An open-source SDK for integrating in-app purchases on iOS
Implement in-app purchases in 30 minutes.
Out-of-the-box back end for iOS in-app purchases
Why choose Adapty SDK?
Correct subscription state at any moment
Rest assured you’ll always get the correct subscriber state across all platforms.
Server-side receipt validation
No need to worry about the correctness and safety of purchase validation.
Handling all kinds of subscription states
Free trials, upgrades, promo offers, family sharing, renewals, and more.
Enterprise-ready platform with a short release cycle
>99.99% SLA reliability and regular product updates.
Configuring platforms
Installing Adapty SDK
Adapty.activate(
"PUBLIC_SDK_KEY",
customerUserId: "YOUR_USER_ID"
)
"PUBLIC_SDK_KEY",
customerUserId: "YOUR_USER_ID"
)
Processing purchasing events
Fast and easy integration
Spend only a couple of hours to integrate the Adapty SDK into your iOS app and we’ll take care of the rest.
Just 5 SDK methods to implement iOS in-app purchases
// Your app's code
import Adapty
Adapty.activate("PUBLIC_SDK_KEY", customerUserId: "YOUR_USER_ID")
// Your app's code
Adapty.getPaywall(placementId: "YOUR_PLACEMENT_ID", locale: "en") { result in
switch result {
case let .success(paywall):
// the requested paywall
case let .failure(error):
// handle the error
}
}
// Your app's code
Adapty.makePurchase(product: product) { result in
switch result {
case let .success(info):
if info.profile.accessLevels["YOUR_ACCESS_LEVEL"]?.isActive ?? false {
// successful purchase
}
case let .failure(error):
// handle the error
}
}
// Your app's code
Adapty.getProfile { result in
if let profile = try? result.get() {
// check the access
}
}
// Your app's code
Adapty.restorePurchases { [weak self] result in
switch result {
case let .success(profile):
if info.profile.accessLevels["YOUR_ACCESS_LEVEL"]?.isActive ?? false {
// successful access restore
}
case let .failure(error):
// handle the error
}
}
Industry leaders choose Adapty
What do you get with Adapty?
Adapty SDK provides tremendous possibilities for growing app revenue
Real-time analytics for your iOS App
Rely on the data with 99.5% accuracy with App Store Connect.
Get startedGravity Fit
Health & Fitness
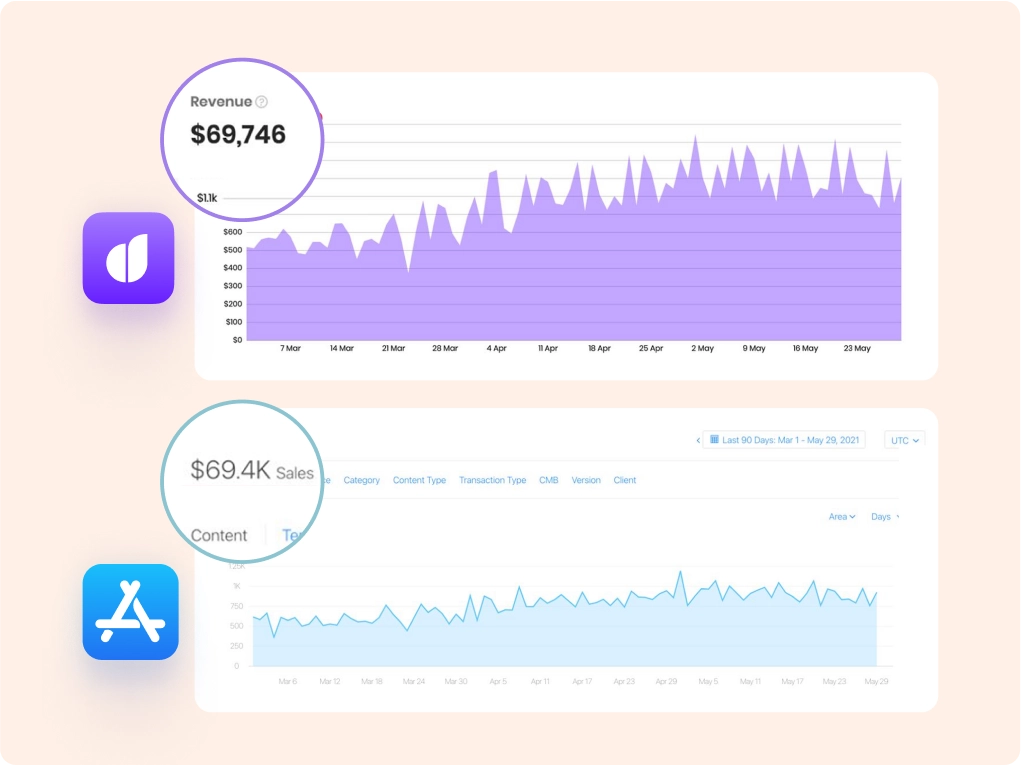
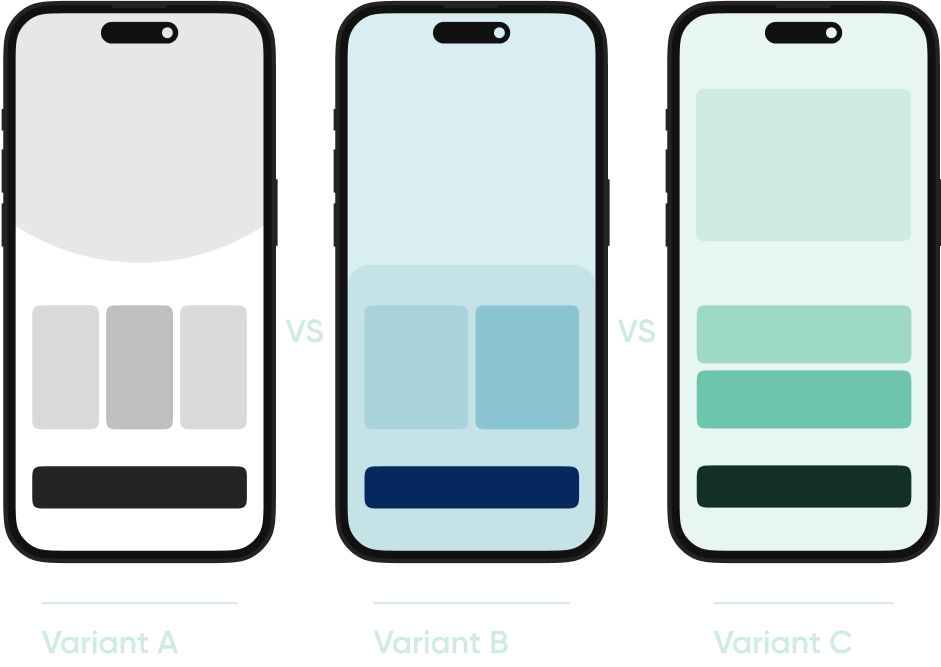
Paywall A/B testing
Find the most profitable paywall and grow your app’s revenue.
Explore A/B testingMentalGrowth
Health & Wellness app
Remote config for paywalls
Change your paywall remotely without having
to re-release the app.
Try it now
Smitten - Dating
Lifestyle
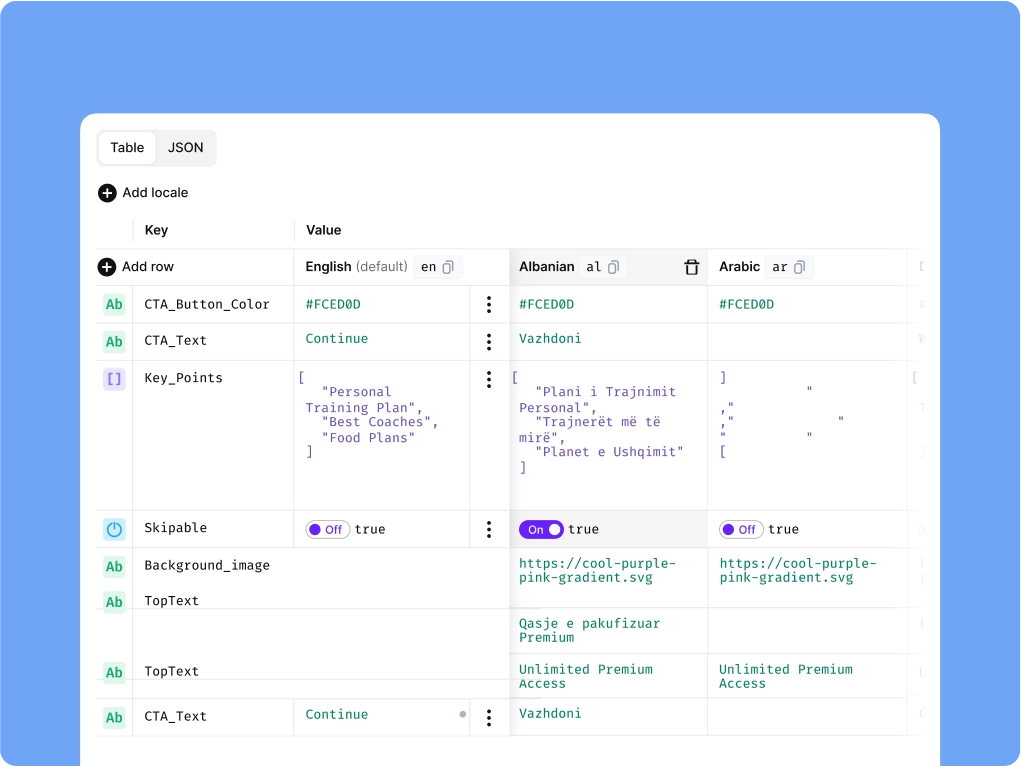